© 2024 fjorge. All rights reserved.
How do I properly use .map(), .filter() and .find() in ECMAScript 2015+?
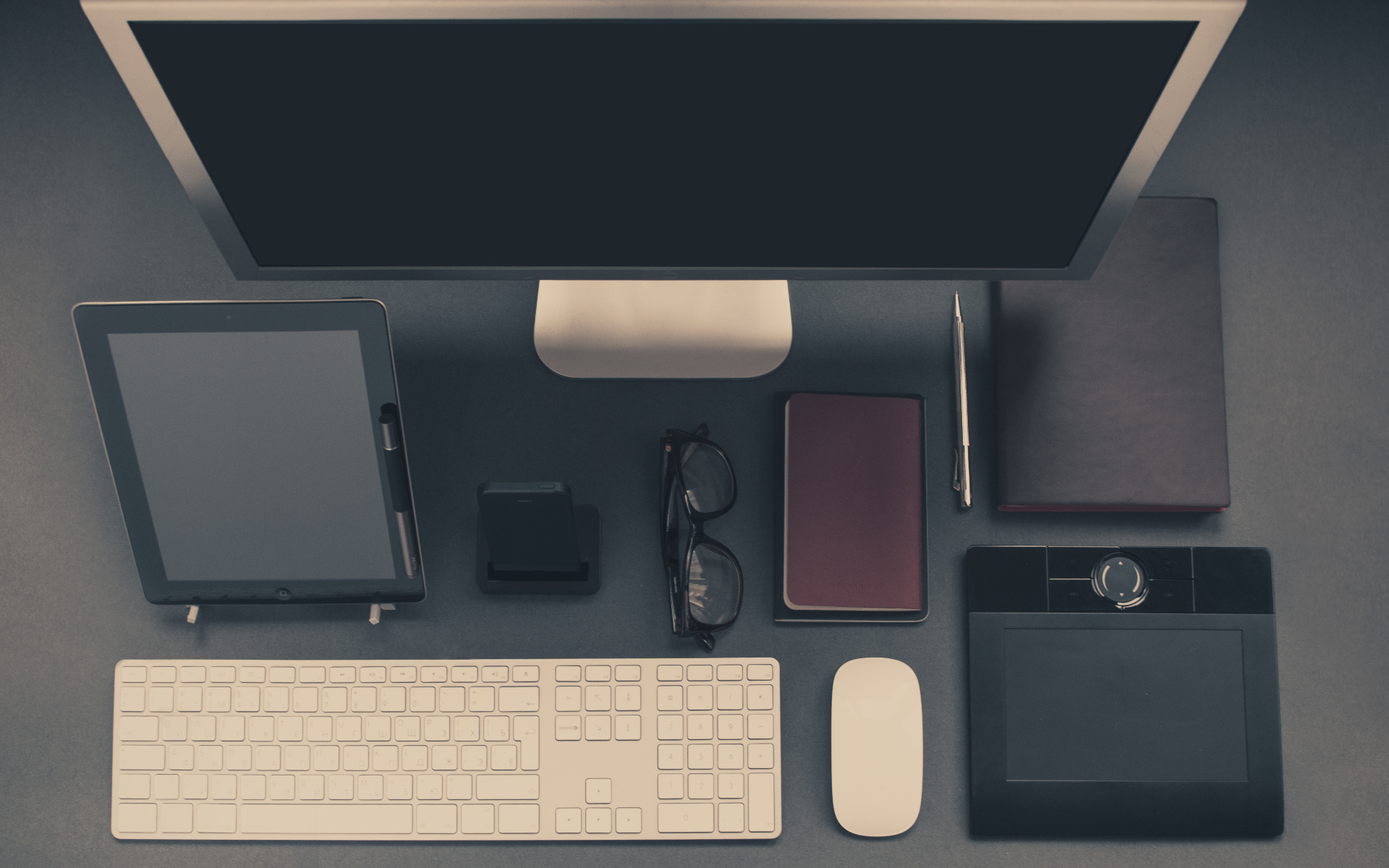
Back in 2015, ES5 introduced .map()
, .filter()
and .find()
methods for iterating over arrays. Yay! Cue confetti cannon. Programming has never been so easy!
That all sounds great, but what does it mean?
This means that you can now iterate over an array in JavaScript and return exactly the results you were looking for. Let's take a look at how each of these methods work:
.map()
.map()
is by far the most familiar method. It can be compared to a for loop. You can loop over an array and for every iteration of an array, you expect to return a result.
let a = [1, 2, 3, "Pikachu", null, 6];
let b = a.map(x => {
return x;
});
console.log(b); // [1, 2, 3, "Pikachu", null, 6]
.filter()
.filter()
takes an array and filters it down to the items in the array that match the conditions you are looking for.
let a = [1, 2, 3, "Pikachu", null, 6];
let b = a.filter(x => {
if (typeof x === 'number') {
return;
}
});
console.log(b); // [1, 2, 3, 6]
.find()
.find()
will iterate over an array until it finds an item that matches the conditions you set and will return that iteratation.
let userId = 101;
let a = [
{id: 100, username: 'johnstamos'},
{id: 101, username: 'bobsaget'},
{id: 102, username: 'davecoulier'},
{id: 103, username: 'ashleyolsen'}
];
let b = a.find(x => x.id === userId);
console.log(b); // [{id: 101, username: 'bobsaget'}]
Pretty cool, huh?
Well, if you thought that was easy, there are plenty of other methods that you could try out when it comes to iterating over arrays in JavaScript. Some other methods to explore are .reduce()
, .every()
, and .forEach()
.