© 2024 fjorge. All rights reserved.
How Do I Create a WordPress Related Posts Section ?
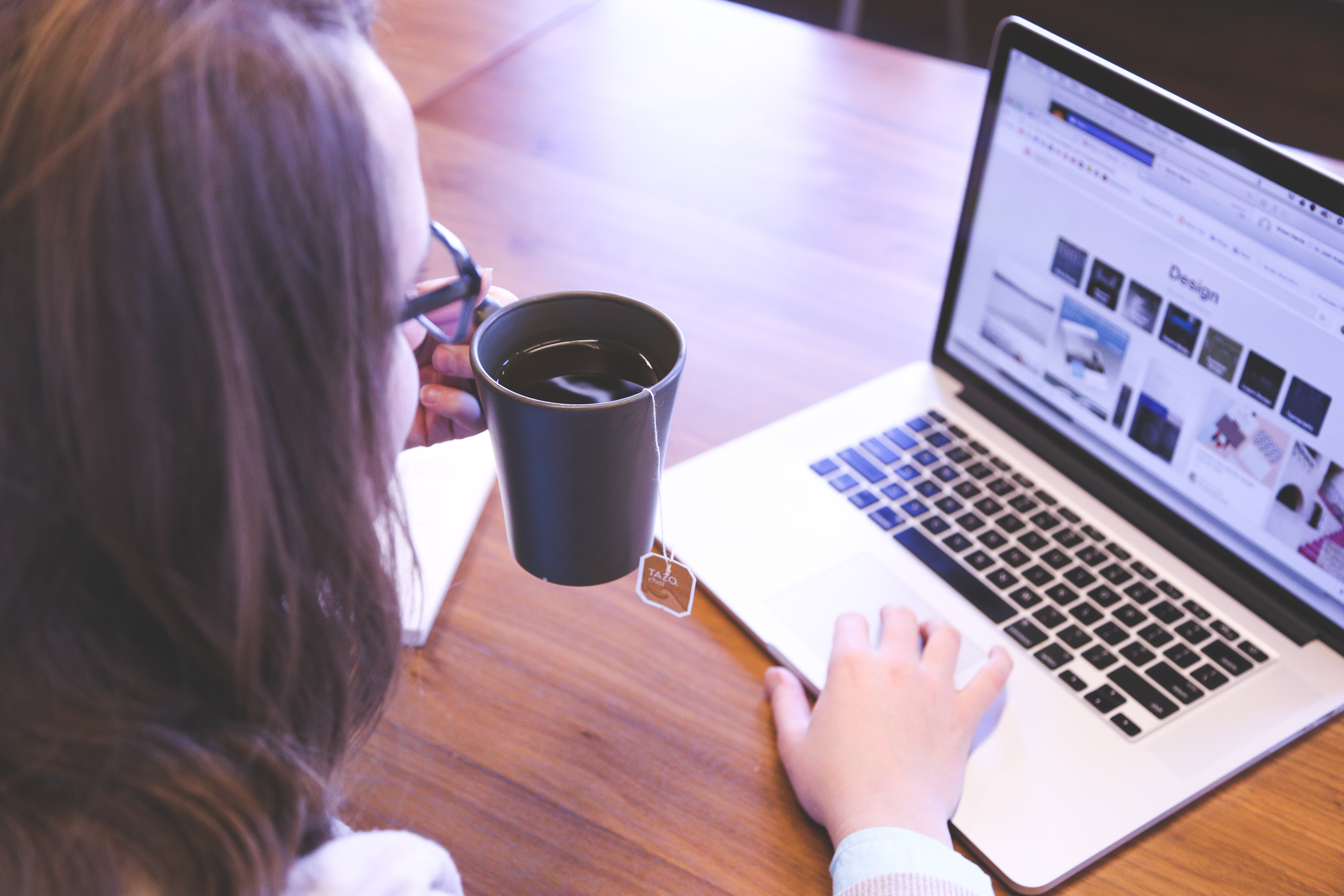
Are you searching for a way to display related blog posts in WordPress? Perhaps they are connected by one or multiple taxonomies? Look no further. The answer lies in a fairly straightforward and flexible WordPress query.
First you want to set up your variables:
$this_post = $post->ID;
$healthtopicTerms = get_the_terms(get_the_ID(), 'health_topic' );
if ($healthtopicTerms){
$healthtopicTerm_list = wp_list_pluck($healthtopicTerms, 'slug' );
}
$categoryTerms = get_the_terms(get_the_ID(), 'category' );
if ($categoryTerms) {
$categoryTerm_list = wp_list_pluck($categoryTerms, 'slug' );
}
In this case, we are assuming that we have two taxonomies: Category and Health Topic. The posts we would like to display are related to one or more terms in either taxonomy. You can update these taxonomies based on your needs.
To grab the terms we need, we use get_the_terms to first get all the terms associated with the current post. Then we use wp_list_pluck to create a list of those term slugs in a format we can easily utilize in the query.
We also have a variable defined to grab the current post ID.
Next, you want to write your query arguments:
$args = array(
'post_type' => 'post',
'posts_per_page' => 3,
'orderby' => 'rand',
'post__not_in' => array($this_post),
'tax_query' => array(
'relation' => 'OR',
array(
'taxonomy' => 'health_topic',
'field' => 'slug',
'terms' => $healthtopicTerm_list,
),
array(
'taxonomy' => 'category',
'field' => 'slug',
'terms' => $categoryTerm_list,
)
)
);
$related_query = new WP_Query( $args ); ?>
Here we have created a query that uses the term list variables from above to list out all terms associated with the current post. We are using an OR relationship so posts will display if the health topic OR category terms are found in the related posts. This could easily be changed to an AND. We are also using post__not_in to exclude the current post we are on.
Finally, we run the loop to display the related posts:
<?php if ( $related_query->have_posts() ) : ?>
<section class="relatedContent">
<?php while ( $related_query->have_posts() ) : $related_query->the_post();?>
<h3><?php the_title(); ?></h3>
<?php the_excerpt(); ?>
<?php endwhile; ?>
</section>
<?php endif; ?>
All together now:
<?php
$this_post = $post->ID;
$healthtopicTerms = get_the_terms(get_the_ID(), 'health_topic' );
if ($healthtopicTerms){
$healthtopicTerm_list = wp_list_pluck($healthtopicTerms, 'slug' );
}
$categoryTerms = get_the_terms(get_the_ID(), 'category' );
if ($categoryTerms) {
$categoryTerm_list = wp_list_pluck($categoryTerms, 'slug' );
}
$args = array(
'post_type' => 'post',
'posts_per_page' => 3,
'orderby' => 'rand',
'post__not_in' => array($this_post),
'tax_query' => array(
'relation' => 'OR',
array(
'taxonomy' => 'health_topic',
'field' => 'slug',
'terms' => $healthtopicTerm_list,
),
array(
'taxonomy' => 'category',
'field' => 'slug',
'terms' => $categoryTerm_list,
)
)
);
$related_query = new WP_Query( $args ); ?>
<?php if ( $related_query->have_posts() ) : ?>
<section class="relatedContent">
<?php while ( $related_query->have_posts() ) : $related_query->the_post();?>
<h3><?php the_title(); ?></h3>
<?php the_excerpt(); ?>
<?php endwhile; ?>
</section>
<?php endif; ?>